Quick overview
In this tutorial you will learn:
- how to setup your html for a parallax website
- how to animate background image at a different speed then page scrolling
- how to precisely control the timing and duration of your animations.
Instructions
Open the
index.html
, /css/main.css
and /js/_main.js
files in your favorite code editor. That’s where we will be doing all our updates. Before we dive in, lets quickly explain what we have for start.Step 1 – Setup
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
| < body > < main > < section id = "slide-1" class = "homeSlide" > < div class = "bcg" > < div class = "hsContainer" > < div class = "hsContent" > < h2 >Simple parallax scrolling is...</ h2 > </ div > </ div > </ div > </ section > <!-- Section 2 --> <!-- Section 3 --> <!-- Section 4 --> </ main > </ body > |
HTML
The code in
The code in
index.html
is pretty simple, we have 4 sections
with a background image .bcg
and a content container .hsContainer
(home slide container) which is by default horizontally and vertically centered.
CSS
Each
Each
section
has a custom background and the content is repositioned to the desired position. The style
of the text is also slightly different on each of the sections, that’s purely to make it nicely readable.
JS
In the
In the
_main.js
we have a simple script which preloads our background images (thanks to ImagesLoaded) and resizes
each of the sections to be100%
of the browser viewport.
If you view the index file in a browser now, you will see 4 sections scrolling as they would normally and the background image
fixed
as defined in our stylesheet. Next we’ll initiate the Skrollr.js and start creating our parallax scrolling effect.Step 2 – Skrollr.js
Add the following lines to your
main.js
to enable Skrollr.js and to refresh it after our sections are resized to 100%
of the height of the viewport.
01
02
03
04
05
| // Init Skrollr var s = skrollr.init(); // Refresh Skrollr after resizing our sections s.refresh($( '.homeSlide' )); |
Section 1
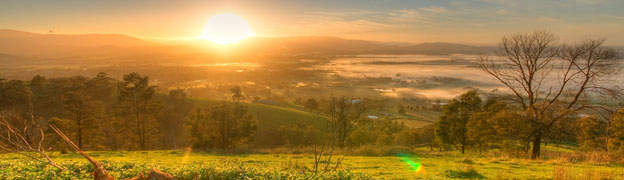
Now we have to add some
data attributes
to our sections to see Skrollr in action and to see our background image and content to move at a different speed. Copy and paste the following lines into your index.html
.
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
| < section id = "slide-1" class = "homeSlide" > < div class = "bcg" data-center = "background-position: 50% 0px;" data-top-bottom = "background-position: 50% -100px;" data-anchor-target = "#slide-1" > < div class = "hsContainer" > < div class = "hsContent" data-center = "bottom: 200px; opacity: 1" data-top = "bottom: 1200px; opacity: 0" data-anchor-target = "#slide-1 h2" > < h2 >Simple parallax scrolling is...</ h2 > </ div > </ div > </ div > </ section > |
What we are telling the browser is to change the background image position from
50% 0
to 50% -100px
, which means that the image is moved up by 100px from the start of the scrolling until the bottom of the #slide-1
hits the top of the browser viewport
.
The text is scrolling with the page until the
#slide-1 h2
hits the centre of our browser and then animates quickly of the screen and fades out, which we have defined in data-top="bottom: 1200px; opacity: 0"
.
The
data-anchor-target
is a very powerful attribute, which enables you to define exactly when should your animation start and finish in relation the defined target
element. Using this attribute correctly enables you to have aprecise control over the timing of your scrolling animations.Section 2
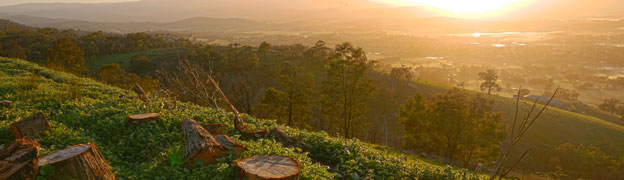
Copy and paste the following lines of code to enable animations on the second slide.
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
| < section id = "slide-2" class = "homeSlide" > < div class = "bcg" data-center = "background-position: 50% 0px;" data-top-bottom = "background-position: 50% -100px;" data-bottom-top = "background-position: 50% 100px;" data-anchor-target = "#slide-2" > < div class = "hsContainer" > < div class = "hsContent" data-center = "opacity: 1" data-center-top = "opacity: 0" data--100-bottom = "opacity: 0;" data-anchor-target = "#slide-2" > < h2 >great for story telling websites.</ h2 > </ div > </ div > </ div > </ section > |
Here we are animating the background image at the same speed to keep things consistent. The difference between #slide-1 and #slide-2 is that the second slide is scrolling through the whole viewport, but the first one loads already visible.
That’s why we are defining both
data-top-bottom
and data-bottom-top
.data-center
is actually not doing anything as it’s value is exactly half way between top and bottom, but experiment with different values and see how the data-center affects the whole animation.
Think about it as another keyframe.
We are going to slightly tweak our css for the
.hsContent
and h2
of this slide, because we want this heading to be fixed to the bottom of the viewport. Update your css for Slide 2 with the following styles.
01
02
03
04
05
06
07
08
09
10
| /* Slide 2 */ #slide -2 .bcg { background-image : url ( '../img/bcg_slide-2.jpg' )} #slide -2 .hsContent { margin-left : -450px ; top : auto ; } #slide -2 h 2 { position : fixed ; top : 70% ; } |
Now the heading is fixed
70%
from the top and doesn’t scroll with the page. This enables us to make this heading visible much earlier than before when it wasn’t fixed. We are also fading it out when the #slide-2
is 100px
from the bottom of the browser.Section 3
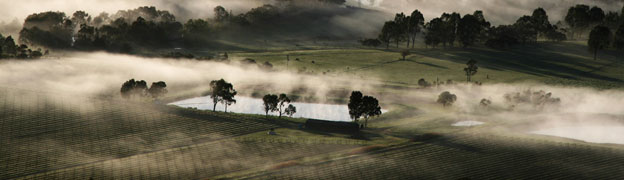
You should know by now what to do…copy and paste the following lines of code into your
index.html
.
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
20
| < section id = "slide-3" class = "homeSlide" > < div class = "bcg" data-center = "background-position: 50% 0px;" data-top-bottom = "background-position: 50% -100px;" data-bottom-top = "background-position: 50% 100px;" data-anchor-target = "#slide-3" > < div class = "hsContainer" > < div class = "hsContent" data--50-bottom = "opacity: 0;" data--200-bottom = "opacity: 1;" data-center = "opacity: 1" data-200-top = "opacity: 0" data-anchor-target = "#slide-3 h2" > < h2 >Now go and create your own story</ h2 > </ div > </ div > </ div > </ section > |
Again our background is animating at the same speed as the rest of the page, which makes things nicely consistent. You can tweak the settings to whatever you like, just keep in mind that subtle parallax scrolling effects usually work much better and are easier on eyes than quick animations.
Section 4
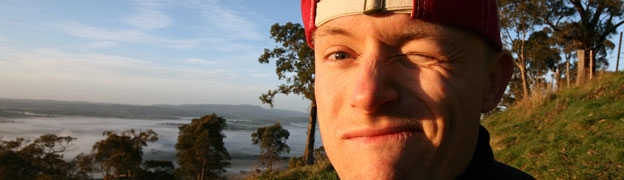
Hey you, still following? Lets copy and paste settings for our last slide.
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
| < section id = "slide-4" class = "homeSlide" > < div class = "bcg" data-center = "background-position: 50% 0px;" data-top-bottom = "background-position: 50% -100px;" data-bottom-top = "background-position: 50% 100px;" data-anchor-target = "#slide-4" > < div class = "hsContainer" > < div class = "hsContent" data-bottom-top = "opacity: 0" data-25p-top = "opacity: 0" data-top = "opacity: 1" data-anchor-target = "#slide-4" > < h2 >and share mine.</ h2 > </ div > </ div > </ div > </ section > |
For the final slide we are also going to slightly tweak our css. We want the text to be
fixed
to the top of the browser and we will remove the background color and padding. Update your stylesheet with the following highlighted lines of code.
01
02
03
04
05
06
07
08
09
10
11
12
| /* Slide 4 */ #slide -4 .bcg { background-image : url ( '../img/bcg_slide-4.jpg' )} #slide -4 .hsContent { margin-left : -450px ; position : fixed ; top : 250px ; } #slide -4 h 2 { background : none ; padding-left : 0 ; padding-right : 0 ; } |
This makes the heading on the last slide fixed to
250px
from the top. The skrollr attributes for .hsContent
are making the heading invisible until thetop of #slide-4
is 25% from the top
of the browser window.Step 3 – Force Height
If you have followed the tutorial and got to this point, you will see everything working fine in the browser and all our parallax scrolling animationsworking nicely. There is only one issue. When you scroll past the last slide, you will see a big black slide coming from nowhere.
Skrollr is by default manipulating the height of the document to make sure all animations fit inside and the black color is our background color.
But what we want is the page to finish at the end of the last slide. To do that we will need to turn off the
forceHeight
option for Skrollr.
Update your
main.js
with the following code.
01
02
03
04
| // Init Skrollr var s = skrollr.init({ forceHeight: false }); |
Now everything should be working exactly how we wanted.
Conclusion
There you have it, a simple parallax scrolling tutorial with a subtle animations. Let me know what do you think about parallax scrolling animations. Are they being overused or used on wrong projects?
The true is, if they are done correctly they can create a visually stunning effects and increase the user engagement. If you have enjoyed this tutorial you will love reading “How to create a parallax scrolling website“.
i dont think i understand about attribute on hsContent, thats confusing me, would you make another tutorial for those attribute? thx
ReplyDeleteI know skrollr works on iphone but the demo does not.
ReplyDeleteCan you explain why .. and do you know how to get skrollr working in wordpress?